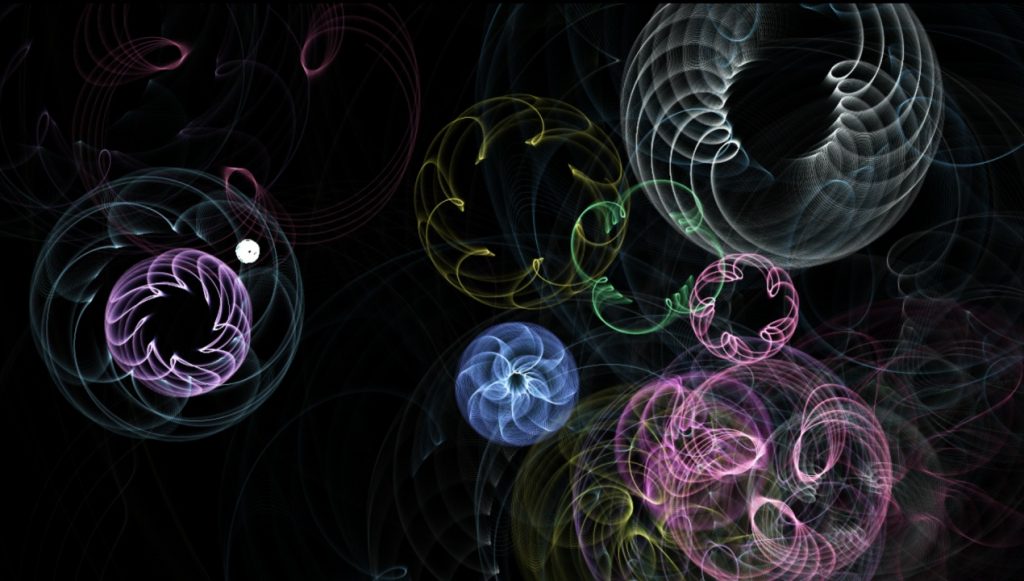
This 1K intro was made for the LoveByte 2025 high-end 1K intro competition, it placed 4th.
The effect is a particle effect based on the spirograph toy I had as a kid. The music is again procedual floatbeat on the GPU, but this time I wanted to see if I could add explicit synchronisation between the visuals and the music. It works, but takes quite some bytes so the visuals couldn’t be very complex. Also, the first time the shader is compiled, the intro might get out of sync. So best to press escape and restart it to be sure sync is as it is supposed to be…
You can download the intro from here: Spirotranquil by Fulcrum
Beware, Windows Defender is once again paranoid and likes to delete the .exes as soon as it sees them. Best to unpack in a folder you’ve excepted from the AV.